Writing WindnTrees View (CRUDSList)
CRUDSList consisting of array of CRUDViews will look like following:
var crudsList = CRUDSList({
'cruds': [
new CRUDView({
'name': 'categories', 'title': 'Categories',
'uri': '/category',
'observer': new CRUDObserver({ 'contentType': new Category({}), 'messages': intialize(new MessageRepository()) })
}),
new CRUDView({
'name': 'ratings', 'title': 'Ratings',
'uri': '/rating',
'observer': new CRUDObserver({ 'contentType': new Rating({}), 'messages': intialize(new MessageRepository()) })
}),
new CRUDView({
'name': 'skill-levels', 'title': 'Skill Levels',
'uri': '/skilllevel',
'observer': new CRUDObserver({ 'contentType': new SkillLevel({}), 'messages': intialize(new MessageRepository()) })
})
],
'observer': new ObjectObserver({})
});
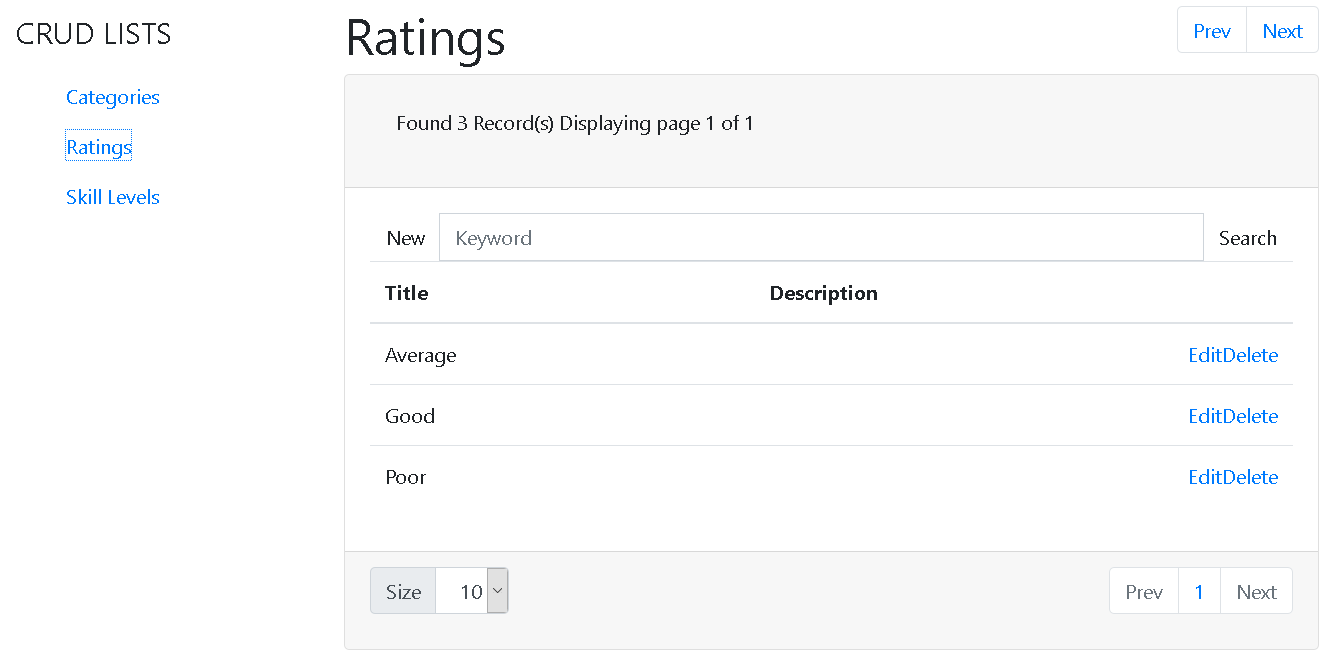